The 3D Utilities are a java library and set of tools for the loading, viewing, manipulating, and comparison of 3D content from among the many 3D formats that exist today. Though a variety of data structures exists to represent 3D data we build everything on a single simple polygonal mesh data structure allowing most users to deal with the loaded 3D data fairly easily from within their custom applications. In addition, being a fairly low level representation of a 3D surface most other 3D representations can be converted to this representation while the inverse can often be non-trivial.
By fixing our 3D data representation we provide a uniform means of constructing a library of file loaders, model viewers, manipulation methods, and comparison measures. Of particular importance is the effort to construct a large open library of 3D file loaders. To our knowledge there does not exist an active open source library for loading 3D data. Java 3D attempted to provide such a functionality some time ago by providing a “Loader” interface by which developers could construct new file loaders. Over the years however very few loaders have been made and distributed. It is possible that the complexity of the very flexible scene graphs used by Java 3D made it a hindrance for all but the more advanced programmers to attempt constructing file loaders. Coupled with the effort required to construct loaders for the many file formats available, many of which being propriety with closed specifications, likely did not help the situation.
It is this projects hope that by “keeping things simple” and imposing this simple mesh data structure we can succeed where others have failed. We hope to encourage programmers at large, both advanced and novice, to contribute file loaders. At the same time we hope to provide a free means of loading a large numbers of 3D formats to developers (in particular new programmers, students, who often re-implement a simple loader to incorporate models in their projects). By developing the library in Java we hope to provide this functionality across platforms and over the web.
In this document we describe the 3D Utilities API including the “Mesh” data structure, the various “ModelViewer” classes implemented to display the loaded models, the “MeshLoader” class to load 3D files into meshes, and the “MeshSignature” used to compare two meshes. We also describe various included tools such as the “ModelBrowser” used to view an manipulate 3D files in the local file system.
# Library
For the full details of what is available in the 3D Utilities library one should refer to the java documentation. In this document we will give a brief overview of the library and how to use it. Note, all classes described below exist within the package “edu.ncsa.model”.
## The Mesh Class
At its heart the “Mesh” class is nothing more than a set of points on the surface of a 3D object and a set of polygonal faces connecting groups of these points together. The points, represented by the “Point” helper class in “edu.ncsa.model.MeshAuxiliary” can be allocated as follows:
```java
Point point = new Point(0.0, 0.0, 0.0);
```
and can be accessed as follows:
```java
System.out.println(point.x + “, “ + point.y + “, “ + point.z);
```
Faces, represented by the “Face” helper class in “edu.ncsa.model.MeshAuxiliary” can be allocated for triangles as follows:
```java
Face face = new Face(0, 1, 2);
```
where the arguments are the indices of vertices in a list of points (with indices starting at 0). The point indices used in the face can then be accessed as follows:
```java
for(int i=0; i vertices = new Vector();
Vector faces = new Vector();
/**
Code to fill in vertices and faces vectors.
**/
Mesh mesh = new Mesh();
Mesh.setVertices(vertices);
Mesh.setFaces(faces);
```
The vertices and faces of a mesh can be later accessed with the “getVertices” and “getFaces” method as follows:
```java
for(int i=0; i ModelConvert file.abc file.xyz
```
The above command loads the “file.abc” file using the “MeshLoader_ABC” loader into a “Mesh” object then through the “MeshLoader_XYZ” loader's save method exports the model to “file.xyz”. Note, the 3D Utilities folder must be in your path for the “ModelConvert” command to be found. In order for this converter to function there must be corresponding loaders with the required functionality implemented.
## Model Viewer Applet
To display 3D content on web pages we have created the “ModelViewerApplet” class which wraps the JOGL “ModelViewer” class as an applet. Using this and the very basics for loading, displaying, and manipulating wavefront *.obj files we provide a java archive “ModelViewerLite.jar” that is created from the projects build file. We recommend using this jar as opposed to the full “3DUtilities.jar” which contains everything, as this will need to be downloaded in the background as users view web pages which utilize its functionality and thus it is desirable to make the file as small in size as possible.
An example of how to call and use the applet can be found in the ModelViewer.php file. The output of this is shown in the figure below. You can call this PHP script directly from a web browser by installing a web server on your machine, copying ModelViewerLite.jar and ModelViewer.php to the public directory and from your browser going to:
```
http://localhost/ModelViewer.php?file=file.obj&width=600&height=600
```
to load the file “file.obj”. Note, this assumes you have a wavefront file, "file.obj", is in that same public directory.
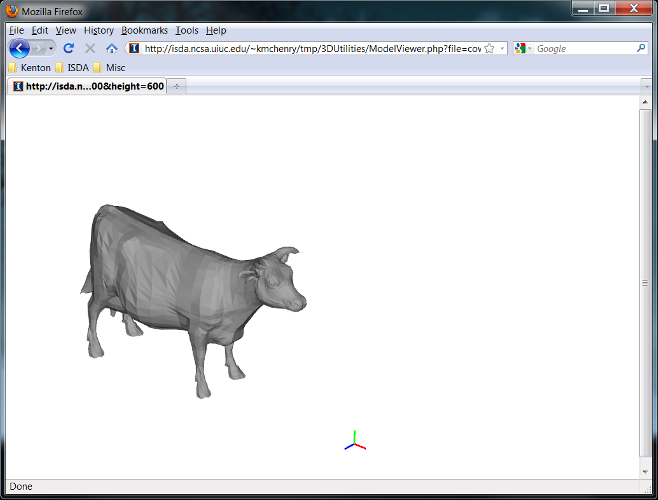
## Model Browser
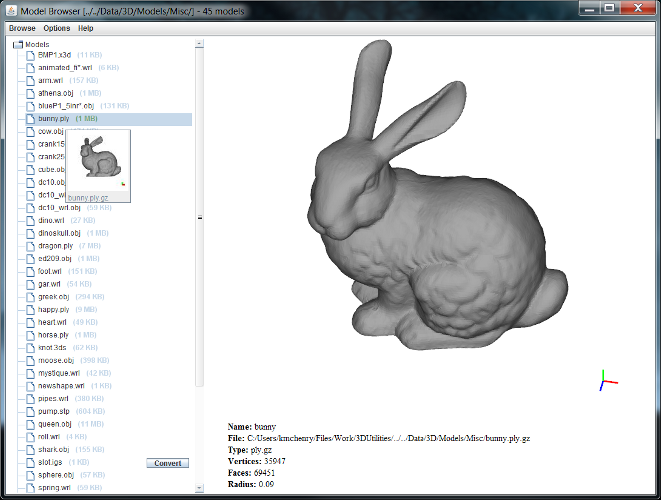
The “ModelBrowser” utility allows a user to see all 3D models found by searching recursively beneath a selected folder (shown in the figure above). The found 3D models, displayed in the left panel, can be selected and viewed/manipulated in the right area through an embedded “ModelViewer” panel. Additional features include: the ability to create image thumbnail images of all found 3D models, the ability to filter out specific file types, and the ability to select multiple 3D files simultaneously and compare them both visually and quantitatively via a selected “MeshSignature” (shown in the figure below).
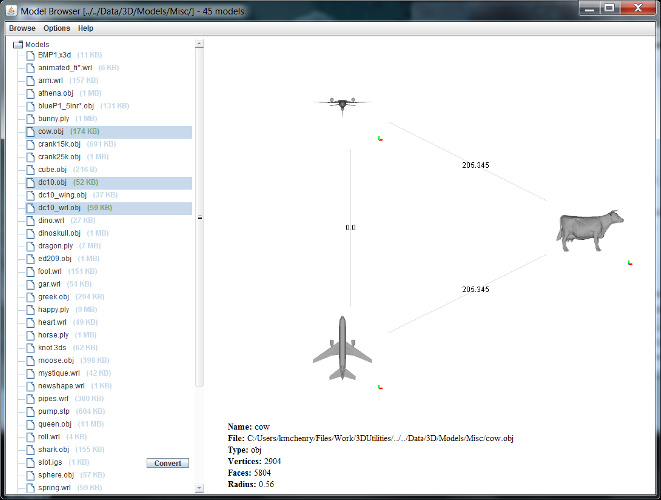
You can run the tool on Windows with the “ModelBrowser.bat” file and on Mac/Linux with the “ModelBrowser.sh” file. Before running the tool you should configure it by editing the files “ModelViewer.ini” and “ModelBrowser.ini”. The parameters in “ModelViewer.ini” are as follows:
* **LoadPath**
* The default path to use when loading new models from the right mouse button popup menu.
* **ExportPath**
* The path to export files to when “Export” is selected from the right mouse button popup menu.
* **MetaDataPath**
* The path to store metadata in such as signatures and thumbnails.
* **Signature**
* The signature to use. Should be set to the fully qualified name of the class including the package (e.g. “edu.ncsa.model.signatures.MeshSignature_lightField”)
* **RebuildSignatures**
* True if signatures should be rebuilt each time the model is loaded. If false the signature will be constructed only the first time the model is loaded.
* **DefaultModel**
* The default model to load. This model will be shown when the model viewer first starts.
* **Adjust**
* True if the loaded model should be scaled and centered to fit in the window. If false the model will be loaded as is in the file.
The parameters in “ModelBrowser.ini” are as follows:
* **LoadPath**
* The default path to use when loading new models from the right mouse button popup menu.
* **MetaDataPath**
* The path to store metadata in such as signatures and thumbnails.
* **Polyglot**
* The URL to a Poyglot web server. The Polyglot server will be used to convert files that have no supporting loaders into a format that is supported.
* **ConvertableList**
* A file containing a line separated list of file extensions that can be converted to a loadable format using the above set Polyglot service.
* **TransparentPanels**
* True if split pane panels should be transparent. If set to transparent then viewed models in the model viewer pane when translated or zoomed sufficiently will be visible behind the left file pane and behind the bottom file data pane.
* **WiiMote**
* True if Wii-mote support should be enabled. A connected Wii-mote can be used to rotate, translate, and zoom into the model within the model viewer.
* **RebuildThumbs**
* True if thumbnails should be rebuilt. If false the thumbnails will only be built if they don’t exist already, otherwise, the previously built thumbnails will be used.